Extract and Visualize Individual Heartbeats#
This example can be referenced by citing the package.
This example shows how to use NeuroKit to extract and visualize the QRS complexes (individual heartbeats) from an electrocardiogram (ECG).
# Load NeuroKit and other useful packages
import neurokit2 as nk
import numpy as np
import matplotlib.pyplot as plt
Extract the cleaned ECG signal#
In this example, we will use a simulated ECG signal. However, you can use any of your signal (for instance, extracted from the dataframe using the read_acqknowledge().
# Simulate 30 seconds of ECG Signal (recorded at 250 samples / second)
ecg_signal = nk.ecg_simulate(duration=30, sampling_rate=250)
Once you have a raw ECG signal in the shape of a vector (i.e., a one-dimensional array), or a list, you can use ecg_process() to process it.
Note: It is critical that you specify the correct sampling rate of your signal throughout many processing functions, as this allows NeuroKit to have a time reference.
# Automatically process the (raw) ECG signal
signals, info = nk.ecg_process(ecg_signal, sampling_rate=250)
This function outputs two elements, a dataframe containing the different signals (raw, cleaned, etc.) and a dictionary containing various additional information (peaks location, …).
Extract R-peaks location#
The processing function does two important things for our purpose: 1) it cleans the signal and 2) it detects the location of the R-peaks. Let’s extract these from the output.
# Extract clean ECG and R-peaks location
rpeaks = info["ECG_R_Peaks"]
cleaned_ecg = signals["ECG_Clean"]
Great. We can visualize the R-peaks location in the signal to make sure it got detected correctly by marking their location in the signal.
# Visualize R-peaks in ECG signal
plot = nk.events_plot(rpeaks, cleaned_ecg)
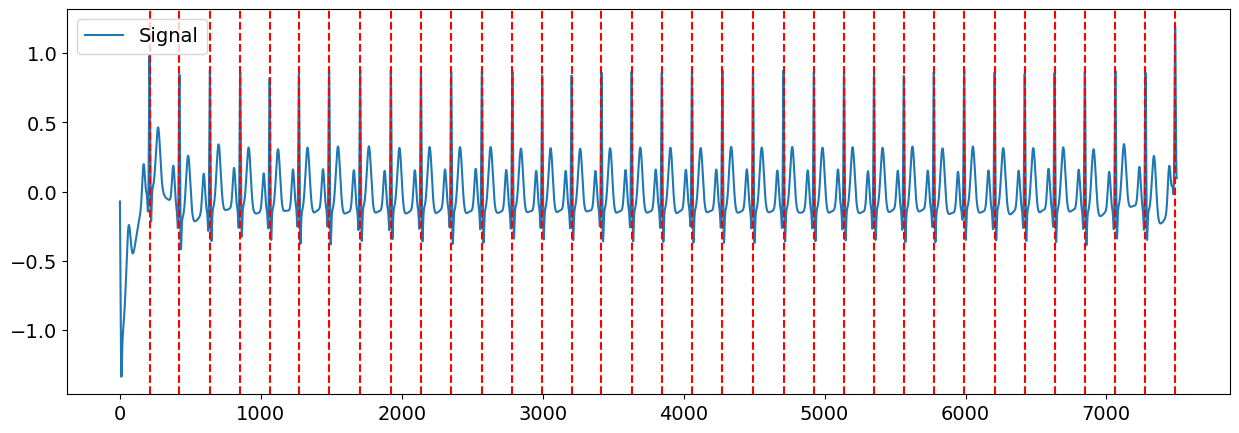
Once that we know where the R-peaks are located, we can create windows of signal around them (of a length of for instance 1 second, ranging from 400 ms before the R-peak), which we can refer to as epochs.
Segment the signal around the heart beats#
You can now epoch all these individual heart beats, synchronized by their R peaks with the ecg_segment() function.
# Plotting all the heart beats
epochs = nk.ecg_segment(cleaned_ecg, rpeaks=None, sampling_rate=250, show=True)
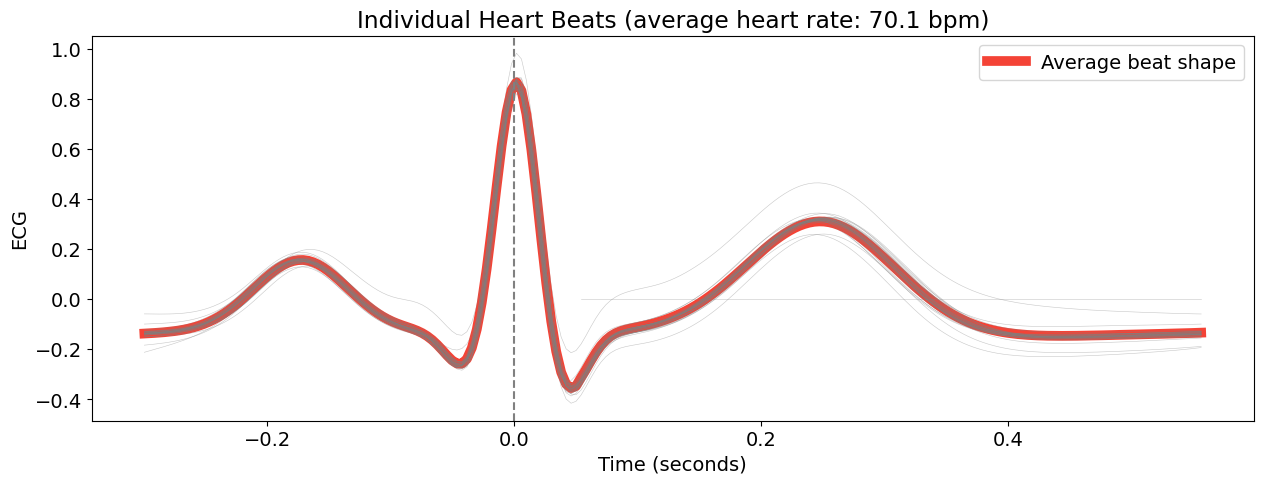
This create a dictionary of dataframes for each ‘epoch’ (in this case, each heart beat).
Advanced Plotting#
This section is written for a more advanced purpose of plotting and visualizing all the heartbeats segments. The code below uses packages other than NeuroKit2 to manually set the colour gradient of the signals and to create a more interactive experience for the user - by hovering your cursor over each signal, an annotation of the signal corresponding to the heart beat index is shown.
Custom colors and legend#
Here, we define a function to create the epochs. It takes in cleaned
as the cleaned signal dataframe, and peaks
as the array of R-peaks locations.
# Define a function to create epochs
def extract_heartbeats(cleaned, peaks, sampling_rate=None):
heartbeats = nk.epochs_create(cleaned,
events=peaks,
epochs_start=-0.3,
epochs_end=0.4,
sampling_rate=sampling_rate)
heartbeats = nk.epochs_to_df(heartbeats)
return heartbeats
heartbeats = extract_heartbeats(cleaned_ecg, peaks=rpeaks, sampling_rate=250)
heartbeats.head()
Signal | Index | Label | Time | |
---|---|---|---|---|
0 | -0.211780 | 135 | 1 | -0.300000 |
1 | -0.206182 | 136 | 1 | -0.295977 |
2 | -0.200562 | 137 | 1 | -0.291954 |
3 | -0.194876 | 138 | 1 | -0.287931 |
4 | -0.189067 | 139 | 1 | -0.283908 |
We then pivot the dataframe so that each column corresponds to the signal values of one channel, or Label.
heartbeats_pivoted = heartbeats.pivot(index='Time', columns='Label', values='Signal')
heartbeats_pivoted.head()
Label | 1 | 10 | 11 | 12 | 13 | 14 | 15 | 16 | 17 | 18 | ... | 32 | 33 | 34 | 35 | 4 | 5 | 6 | 7 | 8 | 9 |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Time | |||||||||||||||||||||
-0.300000 | -0.211780 | -0.139194 | -0.130864 | -0.136518 | -0.140333 | -0.139476 | -0.132068 | -0.133927 | -0.136884 | -0.138800 | ... | -0.126825 | -0.150537 | -0.099231 | -0.199724 | -0.121202 | -0.150639 | -0.136116 | -0.129960 | -0.143432 | -0.136868 |
-0.295977 | -0.206182 | -0.137950 | -0.129669 | -0.135418 | -0.139379 | -0.139054 | -0.131402 | -0.133262 | -0.136028 | -0.138002 | ... | -0.125963 | -0.149092 | -0.098558 | -0.197615 | -0.120082 | -0.149837 | -0.135740 | -0.129015 | -0.142262 | -0.135981 |
-0.291954 | -0.200562 | -0.136561 | -0.128342 | -0.134086 | -0.138226 | -0.138514 | -0.130641 | -0.132468 | -0.135015 | -0.137023 | ... | -0.124925 | -0.147433 | -0.097765 | -0.195335 | -0.118787 | -0.148906 | -0.135233 | -0.127967 | -0.140871 | -0.134897 |
-0.287931 | -0.194876 | -0.134998 | -0.126819 | -0.132472 | -0.136824 | -0.137814 | -0.129763 | -0.131505 | -0.133802 | -0.135825 | ... | -0.123643 | -0.145509 | -0.096822 | -0.192858 | -0.117276 | -0.147819 | -0.134570 | -0.126770 | -0.139194 | -0.133557 |
-0.283908 | -0.189067 | -0.133216 | -0.125015 | -0.130505 | -0.135119 | -0.136903 | -0.128742 | -0.130320 | -0.132327 | -0.134369 | ... | -0.122039 | -0.143273 | -0.095679 | -0.190155 | -0.115489 | -0.146549 | -0.133717 | -0.125359 | -0.137146 | -0.131897 |
5 rows × 35 columns
# Prepare figure
fig, ax = plt.subplots()
ax.set_title("Individual Heart Beats")
ax.set_xlabel("Time (seconds)")
# Aesthetics
labels = list(heartbeats_pivoted)
labels = ['Channel ' + x for x in labels] # Set labels for each signal
cmap = iter(plt.cm.YlOrRd(np.linspace(0,1, int(heartbeats["Label"].nunique())))) # Get color map
lines = [] # Create empty list to contain the plot of each signal
for i, x, color in zip(labels, heartbeats_pivoted, cmap):
line, = ax.plot(heartbeats_pivoted[x], label='%s' % i, color=color)
lines.append(line)
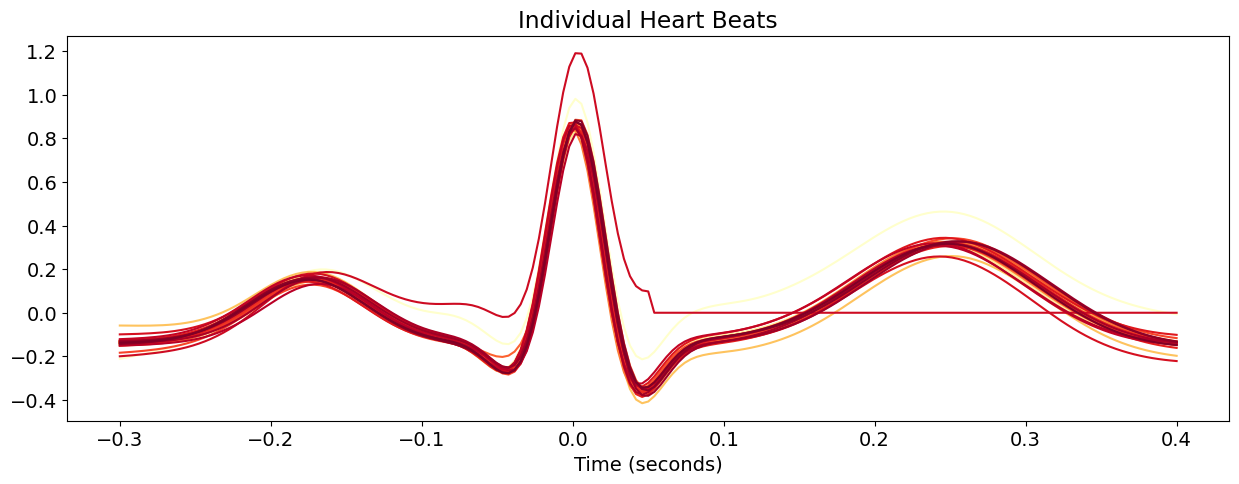
Interactivity#
This section of the code incorporates the aesthetics and interactivity of the plot produced. Unfortunately, the interactivity is not active in this example but it should work in your console! As you hover your cursor over each signal, annotation of the channel that produced it is shown. You will need to uncomment the code below.
Note: you need to install the mplcursors
package for the interactive part (pip install mplcursors
)
# # Import packages
# import ipywidgets as widgets
# from ipywidgets import interact, interact_manual
# import mplcursors
# # Obtain hover cursor
# mplcursors.cursor(lines, hover=True, highlight=True).connect("add", lambda sel: sel.annotation.set_text(sel.artist.get_label()))
# # Return figure
# fig